The Arduino is a fantastic little piece of hardware. The little Atmega microcontroller under the hood is capable of some amazing stuff! In this tutorial, we’re going to learn how to convert a string to an Integer on the Arduino! And in the case that you are using a character array, we’ll also learn how to covert those to an integer!
Sometimes we get data input from a text box on a web page, from a database, or even over the I2C bus from a Raspberry Pi, or some other device. That data is often a number, but delivered to us as a string. When that happens, we will need to convert it to an integer in order to be able to use it for computational uses.
You cannot perform arithmetic on a string. So let’s learn how to deal with this problem!
Convert a String to an Integer
Let’s start with converting a string to an Integer. To do this, we use .toInt() method of the String() function. The code works like this:
myInt = myString.toInt();
Used in a full sketch, we will convert a string to an integer, and then add 1 to it every second.
/*
* CONVERTING A STRING TO AN INTEGER: STRING.TOINT()
* By: TheGeekPub.com
* More Arduino Tutorials: https://www.thegeekpub.com/arduino-tutorials/
*/
String myString = "12345"; //create a string a populate it with "12345"
int myInt; // our integer
void setup() {
Serial.begin(9600); //turn on serial console
myInt = myString.toInt(); //convert string to int
Serial.println(myInt); //print the integer
}
void loop() {
//this loop will add 1 to our integer every second and show in on the screen
delay(1000);
myInt++;
Serial.print("Adding +1: ");
Serial.println(myInt);
}
On the serial console you should see the following output:
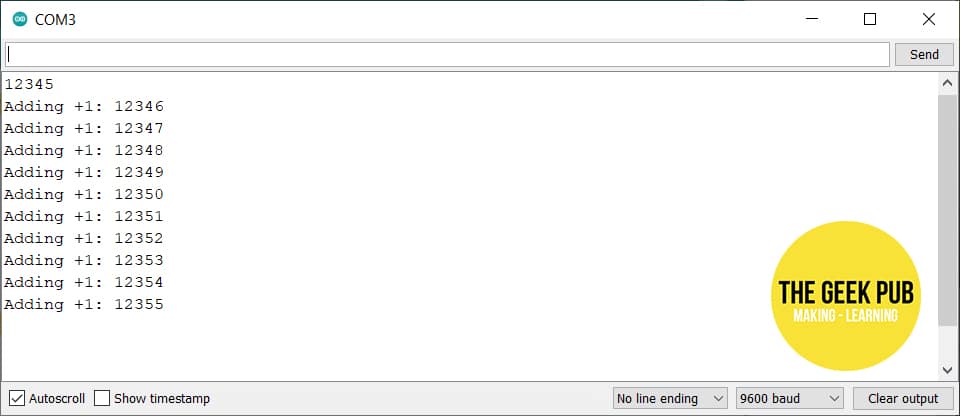
Convert a Character Array to an Integer
Sometimes instead of converting a string to an integer, we will need to convert a character array (char array) to an integer. That can be done using the atoi() function. It stands for “Array to Integer”.
The code looks like this at its basics.
myInt = atoi(myString);
Updating our previous string sketch, the character array sketch would look like this. Same fire drill. We convert the character array to an integer, and then our loop() will add +1 to the number every second.
/*
* CONVERTING A CHARACTER ARRAY TO AN INTEGER: ATOI()
* By: TheGeekPub.com
* More Arduino Tutorials: https://www.thegeekpub.com/arduino-tutorials/
*/
char myString[6] = "12345"; //create an array a populate it with "12345"
int myInt; // our integer
void setup() {
Serial.begin(9600); //turn on serial console
myInt = atoi(myString); //convert char array to int
Serial.println(myInt); //print the integer
}
void loop() {
//this loop will add 1 to our integer every second and show in on the screen
delay(1000);
myInt++;
Serial.print("Adding +1: ");
Serial.println(myInt);
}
On the Serial Console you should see the following output:
Of course, the opposite of converting a string to an integer is to covert an integer to a string!
Next Steps
Now you can go on to the next tutorial or go back to the tutorial index!